In this section we will create a POST request to user controller to create new User in our Data Base. We will now use new Connection Factory method to crate ConnectionFactory instance. Like below
@Bean public ConnectionFactory connectionFactory() { return MySqlConnectionFactory.from(MySqlConnectionConfiguration.builder() .host("localhost") .port(3306) .username("root") .password("") .database("ExampleDB") .build()); }
In above code snippet we have created instance of ConnectionFactory from MySqlConnectionFactory class .
Creating Request Object
We will create a UserSaveRequest class which will match our JSON POST Request Object so that the incoming JOSN data we get as an object in our controller.
@Data
@AllArgsConstructor
@NoArgsConstructor
@Builder
public class UserSaveRequest {
private String firstName;
private String lastName;
}
Controller Changes for new POST Method
Add a @PostMapping to UserController class as below.
@PostMapping
@ResponseStatus(HttpStatus.CREATED)
public Mono<UserSaveRequest> add(@RequestBody UserSaveRequest userSaveRequest) {
return userService.saveUser(userSaveRequest);
}
Using Repository Default Method
We will use inbuilt save method of ReactiveCrudRepository to save our User Object. This save method has signature as below
<S extends T> Mono<S> save(S var1);
We don’t have to do anything other than passing an object of User class created from incoming request to this method. It will save that object into the data base.
Modifying UserService
Add the save method in UserService interface and implementation in UserServiceImpl class . This method is simple it will take the object of UserSaverRquest and create new Object of User from it and then call save method of Repository.
public interface UserService {
Flux<UserResponse> getAllUsers();
Flux<User> findUserByFirstName(String firstName);
Mono<UserSaveRequest> saveUser(UserSaveRequest userSaveRequest);
}
@Override
public Mono<UserSaveRequest> saveUser(UserSaveRequest userSaveRequest) {
User user = new User();
user.setFirstName(userSaveRequest.getFirstName());
user.setLastName(userSaveRequest.getLastName());
return userRepository.save(user)
.thenReturn(userSaveRequest)
.doOnError(e -> {
if(e != null) {
throw new RuntimeException("Exception occurred during data base operation");
}
});
}
userRepository() method will save the object to Data base. the .thenReturn() method will get called when Mono is completed successfully and return the same UserSaveRequest object as we are not exposing any of the primary keys of data base to the client of the api. The .doOnError() will throw a RuntimeException if there some error while saving it to data base.
Response From API Call
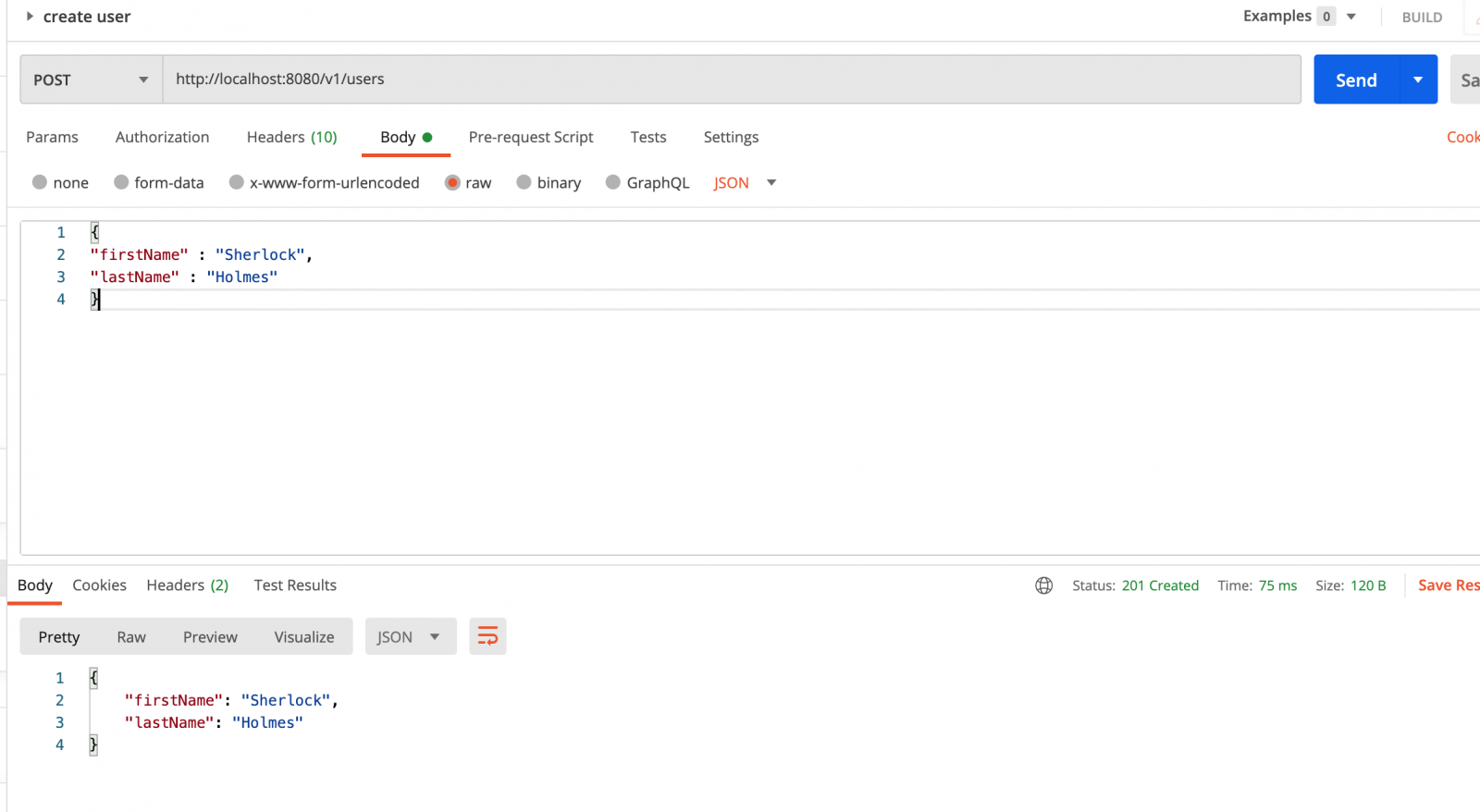
You can check the code commit for this changes here